APIs or Application Programming Interfaces are magical creatures, I mean really; or at least to us, programmers.
Before I tell you more APIs, it's important to first briefly go through "Interfaces" first.
Interfaces are everywhere, in your smartphones as GUI (Graphical User Interfaces) based music player app offering to end users, or BASH shell offering CLI (Command Line Interfaces) to end users and even to programmers.
So what does an Interfaces do? Interfaces adds a layer of abstraction, hiding away the intricate details of what and how something works underneath. The users of interfaces don't need to know how music player app plays the music, or command line user to know how the command gets executed on press of a button.
That means, APIs bring abstraction but to different kind of users, they are not end users like in a music player example, but instead developers or Programmers (for 'P' in API).
For example if we are the programmer of the music player app, we might not need to implement code for gesture or play/pause feature.
This can be provided by an API of an SDK (Software Development Kit) provided by the platform.
You'll also find other libraries offering their APIs to aid in your work, like for e.g. TensorFlow, a mathematical computation framework written in C++ offers it's API in Python and Java as well.
But for most part when we talk about APIs, we are talking about Web APIs, and not an API of a library or an SDK, unless as told.
So, In this article we'll go through all about APIs, what they do, how they do, and what do we need to keep in mind as a developer when building our Web APIs.
What exactly is an API?
API or a Web API is an software interface accessibly via internet which offers a connection between your software and the software running on the server as a type of service.
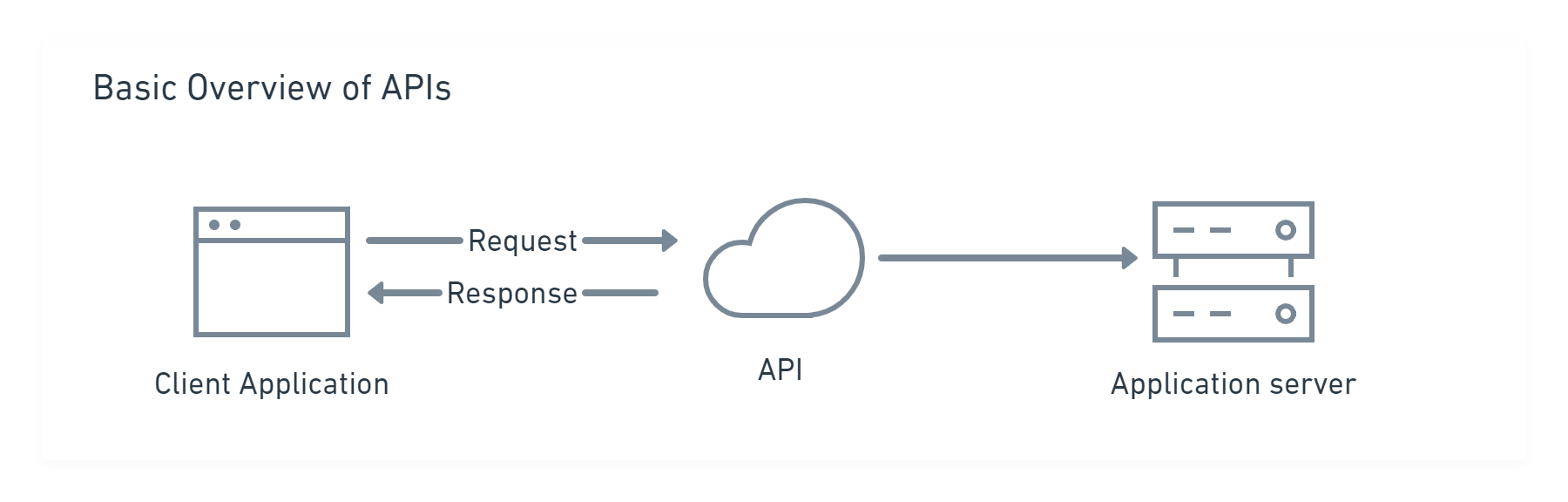
This allows us to perform some operations on the server, or get some data from the server or both; performing operation and getting the result back from the server.
This can be especially useful when:
- The client application needs some kind of service but don't have the capabilities/resources to do it. Say for example, Shazam (a popular music identification app) might need machine learning/Deep Learning services to run which the client side will not have resources to run them because of performance constraints.
- or the service it reside in server and database needs to be accessed for CRUD operations (Create Read Update Delete). Like storing records of a user,
API developer exposes endpoints relative to domain on which the API is hosted where on passing a request to the endpoint, we'll get a response.
So for example, an endpoint might look something like this:
<domain-name>/reply
<domain-name>/posts/reply
- from Spotify
https://api.spotify.com/v1/albums/{id}/tracks
- from twitter
https://api.twiiter.com/2/users/{id}/liked_tweets
Making an HTTP request with a suitable HTTP method to an endpoint gets the process started.
A "Hello World" example of an API
What internally gets done depends upon your imagination. But let's create our own simple API using FastAPI framework in Python.
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
def check_reply():
return {"reply": "hello world"}
Running this app locally, when we visit localhost (http://127.0.0.1/)
, we'll be greeted with a JSON reply.
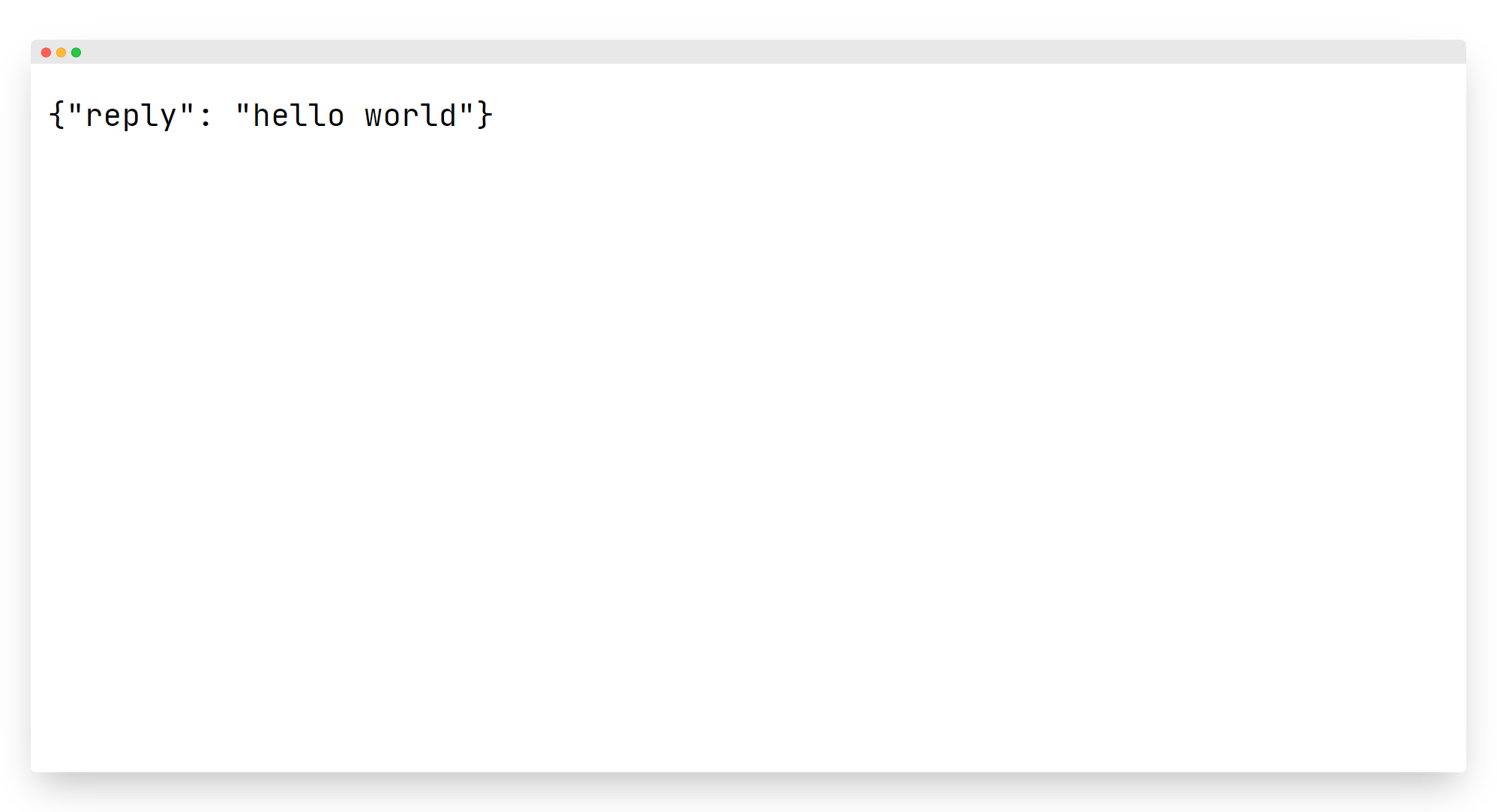
Couple of point to note here:
@app.get("/")
decorator make sure that the method only runs when a request withGET
method is made to/
root URL.- JSON means JavaScript Object Notation, i.e., JavaScript's way of denoting an object. It's very similar to Python Dictionary and that's why we returned a dictionary with a key-value pair which FastAPI make sure to convert to JSON before sending the reply. This object can be anything you like, which could be JSON-ified.
- There is another format in which a reply could be send, that is XML (EXtensible Markup Language) which used to be popular for sending raw text data over the internet before JSON came along.
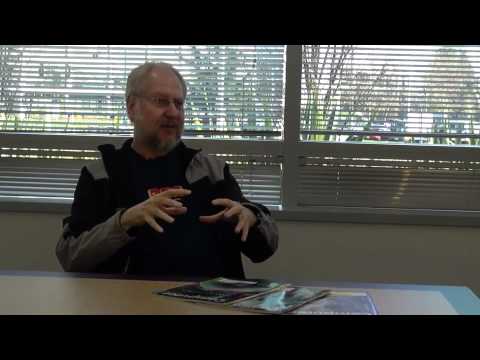
When click on a link of a website, the HTTP request method that our browser creates is GET
method.
And that's why we were able to get a response back.
Now what if instead of just getting some data, we want to upload some data too?
Well that requires us to change the HTTP method from GET
to something like POST
. Let's discuss HTTP methods first and what do they mean to our API.
HTTP Methods
Our API relies completely on HTTP protocol for communication. So which request needs to be deal with in which way is all defined by HTTP.
HTTP request methods indicate the desired action to be performed for a given resource identified by an endpoint.
There are variety of request methods but to us these five are most important when creating our own APIs.
GET
method is used to read (or retrieve) a representation of a resource. Requests usingGET
should only retrieve data. A successful request is returned with an JSON/XML response with HTPP status code of 200 (0k) or in case of an error with 404 (Resource not found) or with 400 (Bad Request)POST
method is most-often utilized to create new resources, by submitting an entity to the specified resource, often causing a change in state or side effects on the server, like creating a new tuple in a database table. On successful creation, return HTTP status 201, returning a Location header with a link to the newly-created resource in a JSON/XML response.PUT
method is used for used for updating/replacing the current representations of the target resource with the request payload. On successfulPUT
request, return 200 (or 204 if not returning any content in the body).PATCH
method applies partial modifications to a resource. The might look similar toPUT
, but inPATCH
request the body contains a set of instructions describing how a resource currently residing on server should be modified to produce a new version instead of just a modified part of resource.DELETE
method deletes the specified resource identified by the URI.
A specific request should only lead to what the request method implies, nothing more, nothing less. For e.g., a GET method shouldn't update a resource, only fetch.
REST – Representational State Transfer
Today most APIs are designed to conform with REST design principles and is the most prominent architecture style that is used to design APIs today. REST is not a protocol, but rather a design philosophy that builds upon the principles of HTTP.
The reason for this dominance is the flexibility and freedom it provides for developers over other options such as SOAP or XML-RPC.
That's why APIs designed with REST in mind are often called RESTful APIs.
The only requirement being that the API needs to be written while abiding to these 6 architectural constraints:
- Client-Server Architecture – The Client and server applications must be completely independent of each other. Client is only supposed to know about the URI the resource is located and can't interact with server application in any form. The server application too can't perform any kind of interaction other that providing the request resource.
- Statelessness – The designed API should be stateless, meaning that each request needs to include all the information necessary for processing it, no server-side sessions.
- Layered System – In REST APIs, the calls and responses go through different layers. So, API need to be designed so that neither the client nor the server can tell whether it communicates with end application or an intermediary
- Cacheability – When possible resources should be cacheable on client or server side. This has to do with performance improvement on client side, while increasing scalability on server side.
- Uniform Design – All API request for same resource should look same, no matter where the request comes from. So a REST API needs to ensure that same piece of data, such as name or email address of a user belongs to only one Uniform Resource Identifier (URI).
- Code on Demand (optional) – REST APIs usually send static resources, but sometimes can also contain executable code, in which case the code should only run on-demand.
SOAP v/s REST
SOAP or Simple Object Access Protocol in contrast to REST is an XML-based protocol for making network API requests. Although it most is most commonly used over HTTP, it aims to be independent from HTTP and avoids using most HTTP features (like HTTP methods).
SOAP had a lot of rules. It comes with a sprawling and complex multitude of related standards that add various features. SOAP web service is described using an XML-based language called the Web Services Description Language, or WSDL.
WSDL is not designed to be human readable, and as SOAP messages are often too complex to construct manually, users of SOAP rely heavily on tool support, code generation and IDEs.
That means, even though SOAP and its various extensions offered standardization, interoperability between different vendors' implementation often causes problems. For these reasons, SOAP fall out of favor and REST grew up.
REST's main idea was for each piece of data the URL should stay the same, but operation would change depending on what method was used. For example request to "https://website.com/cart" with GET will return all cart item, but a POST request to the same URL would add an item to cart.
REST also also offers a greater variety of data formats rather than just sticking to XML, while most APIs default to JSON which offers better support for browser clients, faster parsing and works better with data. This means superior performance, particularly through caching for information that's not altered and not dynamic.
REST services are often described using a definition format such as OpenAPI. Let's save that topic for some other day.
Hope you learned something.
This is Anurag Dhadse, signing off.